• 5 min read
Intersection Observer in React - A Practical Guide
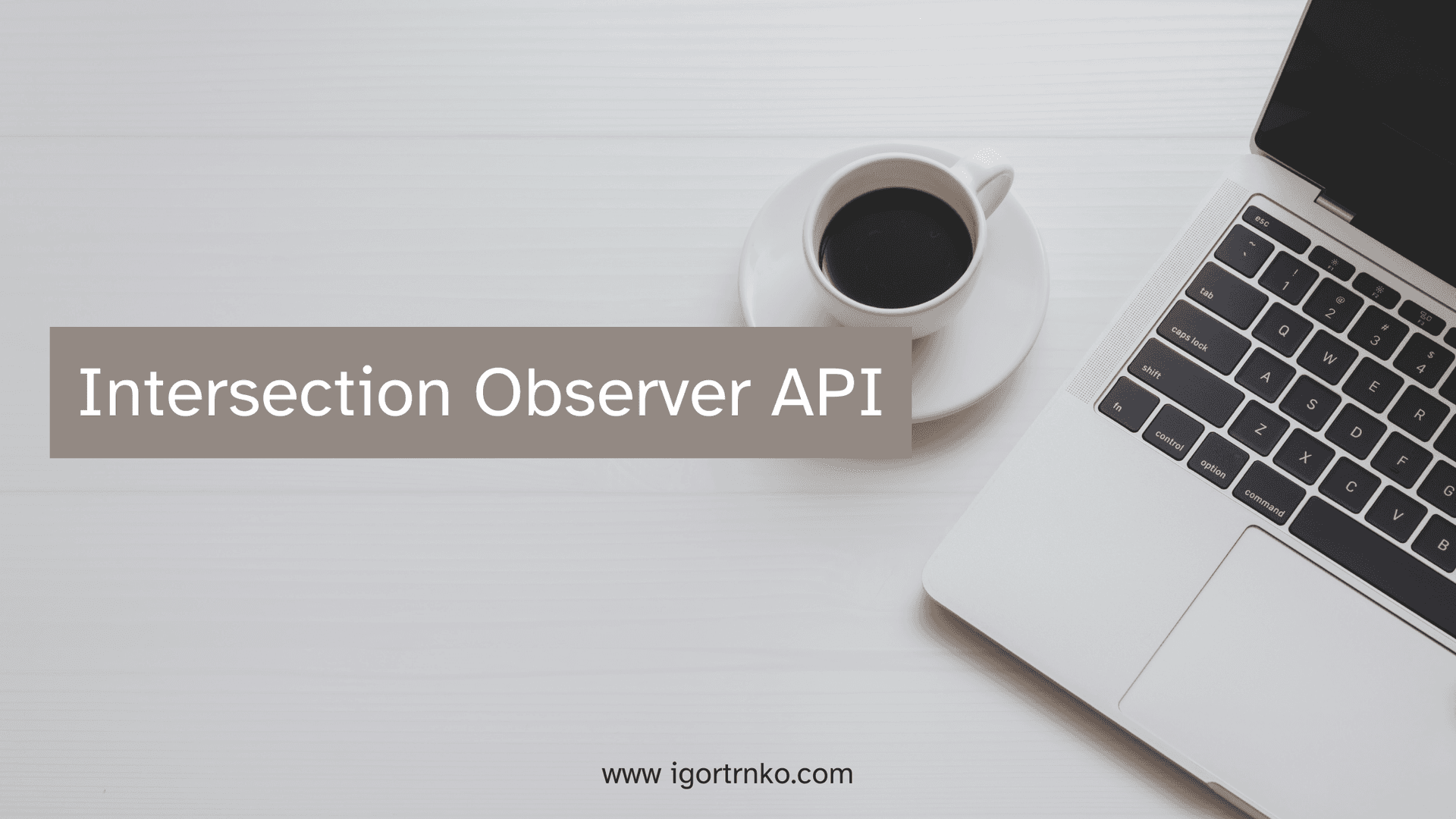
Introduction
The JavaScript Intersection Observer is a powerful tool that allows developers to monitor when an element enters or leaves a specific location on the web. The Intersection Observer API is a browser feature built into modern browsers that makes it easy to use this functionality without the need for complex calculations and event listeners. In this blog post, we will discuss the Intersection Observer API is discussed in detail and we give some examples of its application.
How Intersection Observer Works
The Intersection Observer API works by observing changes in the position of an element relative to a specific viewport or parent element. When an element intersects with the observed area, the Intersection Observer API will fire a callback function that can be used to trigger specific actions. This is especially useful for dealing with lazy loading images and endless web page navigation.
The Intersection Observer API is very flexible and can be configured to handle a variety of applications. It allows developers to define a threshold value that determines how visible an element needs to be before the callback function is triggered. Additionally, developers can specify a parent element to be used as a reference for the checked field.
Example Usage
Let’s take a look at an example of using the Intersection Observer API to create images with lazy loading on the web. First, we need to create the Intersection Observer object and specify options for the observer:
javascript
const observer = new IntersectionObserver((entries, observer) => { entries.forEach(entry => { if (entry.isIntersecting) { const lazyImage = entry.target; lazyImage.src = lazyImage.dataset.src; observer.unobserve(lazyImage); } }); }, { threshold: 0.5 });
In this example, we create a new Intersection Observer object and pass it to the callback function and options object. The callback function accepts two arguments: the set of IntersectionObserverEntry objects and the observer object itself.
Next, we loop through each entry in the array to see if the entry matches the currently checked field. If so, we retrieve the lazy image element and set its "src" attribute to the value of the "data-src" attribute. Finally, we call the "unobserve" method on the observer object to stop observing the element.
To use this Intersection Observer object, we need to specify which objects we want to observe:
javascript
const lazyImages = document.querySelectorAll('.lazy'); lazyImages.forEach(lazyImage => { observer.observe(lazyImage); });
In this example, we use the "querySelectorAll" method to select all the elements of the "lazy" class and then loop through each element and call the "observe" method on the Intersection Observer object.
Custom Hook in React
Let’s create a custom hook in React that will use the Intersection Observer API to check when an element enters the viewport. This hook can be used to implement images with lazy loading or any other task that needs to know when an element is in the scene.
javascript
import { useState, useEffect } from 'react'; const useIntersectionObserver = (ref, options) => { const [isVisible, setIsVisible] = useState(false); useEffect(() => { const observer = new IntersectionObserver(([entry]) => { setIsVisible(entry.isIntersecting); }, options); if (ref.current) { observer.observe(ref.current); } return () => { if (ref.current) { observer.unobserve(ref.current); } }; }, [ref, options]); return isVisible; };
In this example, we can use the useState and useEffect hooks to create a custom hook called useIntersectionObserver. This hook takes two arguments: the ref of the element we want to look at and the options object that specifies the threshold and root elements.
In the useEffect hook we create a new Intersection Observer object and pass in a callback function that updates the isVisible state whenever the observed element moves to or from the viewport and then use the observe and unobserve methods to initialize and we stop looking at the element.
Finally, we return the isVisible condition from the custom hook, which can be used to conditionally render the contents based on whether the observed object is visible or not.
Here is an example of how to use this custom hook in a React component.
javascript
import { useRef } from 'react'; import useIntersectionObserver from './useIntersectionObserver'; const MyComponent = () => { const ref = useRef(null); const isVisible = useIntersectionObserver(ref, { threshold: 0.5 }); return ( <div ref={ref}> {isVisible ? <img src="my-image.jpg" /> : null} </div> ); };
In this example, we create a ref of the div element and pass it into the useIntersectionObserver hook with an options object specifying a threshold of 0.5. We then use the isVisible condition to conditionally render the image in the div element when it enters the viewport.
Conclusion
The JavaScript Intersection Observer is a powerful tool that can be used to implement various functions in web pages. It allows developers to easily control when an element enters or leaves a specific location on the grid, which is especially useful for implementing lazy loading and endless scrolling of images The Intersection Observer API is easy to use and capable change it dramatically, making it a valuable addition to any developer's toolkit. By creating a custom hook in React that uses the Intersection Observer API, we can easily implement this functionality inside our React components.